alert()
This is actually a window object, but I use it all the time. It’s very useful when debugging, or trying to figure out what the heck is going on with your code. It gives you an idea of how the browser/window works—or doesn’t. Mwah ha.
click( )
This one is the most used because you want your site to be interactive. You want users to be able to do stuff and have it effect the site—like with customizable windows, forms, shopping carts. You don’t want you site to be flat and boring.
This example is just your basic alert. When you click on id=”button”, an alert pops up saying “You clicked on a button’. How exciting.
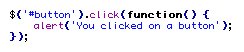
With the next example, when you click on class=”htmlClick”, then three various functions are executed.
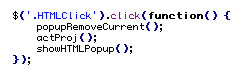
css( )
With css, you can adjust the style of an object using various methods. This one is fun and simple if you already know how to write css.
The grandchildren of this function change the border color from blue to lime green using the hover method (which we’ll talk about soon).
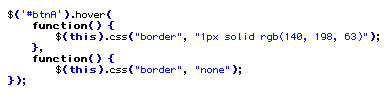
Notice the formatting. jQuery will interpret both of these the same:
.css({“background-color”: “white”, “margin-right”: “15px”})
.css({backgroundColor: “white”, marginRight: “15px”});
CSS notation requires quotation marks because of the hyphen whereas DOM notation does not.
addClass( )/removeClass( )
A more efficient way of handling styles, especially if adding multiple declarations, is to use addClass()/removeClass(). An important thing to note is that you can add as many classes as you want. They will all be applied, and they will stay there until you remove them. The addClass() method does not replace classes!
In this example, I am using ‘show’ and ‘hide’ classes to do exactly as their names imply—to add and show different named elements on the page when I click on #projectsWrapper.

hide( )/show( )
Additionally, you can use these functions to hide/show elements. You can also apply some special effects such as duration, easing, and a function to execute once the element’s completed its parent function. Like alert(‘The object has disappeared. Voila!’);
html( )
This can be used to insert or replace elements within the DOM.
In this example from my bingo game, we’re focusing on the last line. #ball is just a container or div. The two variables ‘letterColumn’ and ‘range’ are defined above in the cases. You can see that the html function inserts the 3 strings, ‘Current Ball:’ + ‘B–‘ + ‘12’. Yay.
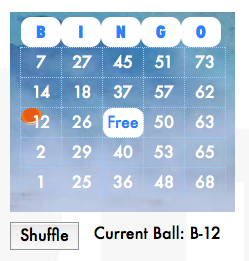
each( )
The each() function loops through elements you want to apply the same effects to. So for example, let’s say you have a list of textual links and you want the background color to change when you hover over it. The each() function will iterate through the lists of links and perform the CSS functions.

hover( )
Looking at the same example as the above each() function, you can see a ‘function waxOn() and function waxOff(). To me, those are pretty meaningful names, but you can name them whatever you want. So when we hover over an element with out mouse, we want something to happen. We want users to know that the links are links, that they’re active. Get the users to feel included in the interactive process. In this example, the background of each link will go from white to silver to white again.

You can also have another function occur, like have a small popup window, have a thumbnail image appear, trigger another event, whatever.
toggle( )
Toggle() is a bit more tricky. You have to play around with this one to properly understand how it works and how it differs from slideToggle. Essentially, toggle is like a switch—on/off. It also has an assumed click, so you don’t surround the function with a click() event.
For example, let’s say we have 3 blue buttons, each with a toggle event. When you click on the button once, it turns green. Click again, it changes to red. Click once more…it goes back to blue. You can add multiple functions with the toggle() event.
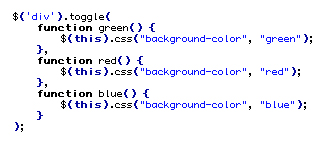